Flaskでとてもシンプルなログインページを実装する方法を紹介します。
環境
- Windows 10 64bit
- Python 3.8.3
- Flask 1.1.1
フォルダ構成
├ app.py
└ templates/
├ login.html
├ welcome.html
└ contents.html
コードの中身
app.py
from flask import Flask, request, redirect, render_template, flash, session
app = Flask(__name__)
app.config[‘SECRET_KEY’] = ‘secret_key’
app.config[‘USERNAME’] = ‘user’
app.config[‘PASSWORD’] = ‘pass’
@app.route(‘/’)
def index():
if “flag” in session and session[“flag”]:
return render_template(‘welcome.html’, username=session[“username”])
return redirect(‘/login’)
@app.route(‘/login’, methods=[‘GET’])
def login():
if “flag” in session and session[“flag”]:
return redirect(‘/welcome’)
return render_template(‘login.html’)
@app.route(‘/login’, methods=[‘POST’])
def login_post():
username = request.form[“username”]
password = request.form[“password”]
if username != app.config[‘USERNAME’]:
flash(‘ユーザ名が異なります’)
elif password != app.config[‘PASSWORD’]:
flash(‘パスワードが異なります’)
else:
session[“flag”] = True
session[“username”] = username
if session[“flag”]:
return render_template(‘welcome.html’, username=session[“username”])
else:
return redirect(‘/login’)
@app.route(‘/welcome’)
def welcome():
if “flag” in session and session[“flag”]:
return render_template(‘welcome.html’, username=session[“username”])
return redirect(‘/login’)
@app.route(‘/contents’)
def contents():
if “flag” in session and session[“flag”]:
return render_template(‘contents.html’, username=session[“username”])
return redirect(‘/login’)
@app.route(‘/logout’)
def logout():
session.pop(‘username’, None)
session.pop(“flag”, None)
session[“username”] = None
session[“flag”] = False
flash(‘ログアウトしました’)
return redirect(“/login”)
if __name__ == ‘__main__’:
app.run(debug=True)
login.html
<!DOCTYPE html>
<html lang=“ja”>
<head>
<meta charset=“utf-8“ />
<meta name=“viewport” content=“width=device-width, initial-scale=1.0” />
<title>ログイン</title>
</head>
<body>
{% with messages = get_flashed_messages() %}
{% if messages %}
{% for message in messages %}
<p>{{ message }}</p>
{% endfor %}
{% endif %}
{% endwith %}
<h1>ようこそ!</h1>
<form action=“/login” method=“post”>
<div>
<label for=“user”>ユーザー名</label>
<input type=“text” id=“user” name=“username”>
</div>
<div>
<label for=“pass”>パスワード</label>
<input type=“password” id=“pass” name=“password”>
</div>
<button type=“submit”>ログイン</button>
</form>
</body>
</html>
装飾のないシンプルなログイン画面です。
welcome.html
<!DOCTYPE html>
<html lang=“ja”>
<head>
<meta charset=“UTF-8“>
<meta name=“viewport” content=“width=device-width, initial-scale=1.0”>
<title>Welcome</title>
</head>
<body>
<h1>Welcomeページ</h1>
<h2>ようこそ! {{ username }}さん</h2>
<p>このページはログインしないと表示されません</p>
<ul>
<li><a href=“{{ url_for(‘contents’) }}”>コンテンツ</a></li>
<li><a href=“{{ url_for(‘logout’) }}”>ログアウト</a></li>
</ul>
</body>
</html>
ログイン後に表示されるページです。
ログインしたときのユーザーIDをrender_templateで渡しています。
ログアウトをクリックするとログイン画面に戻ります。
contents.html
<!DOCTYPE html>
<html lang=“ja”>
<head>
<meta charset=“UTF-8“>
<meta name=“viewport” content=“width=device-width, initial-scale=1.0”>
<title>コンテンツ</title>
</head>
<body>
<h1>コンテンツページ</h1>
<h2>こんにちは! {{ username }}さん</h2>
<p>このページはログインしないと表示されません</p>
<ul>
<li><a href=“{{ url_for(‘welcome’) }}”>Welcomeページへ</a></li>
<li><a href=“{{ url_for(‘logout’) }}”>ログアウト</a></li>
</ul>
</body>
</html>
ログイン後に表示されるページその2です。
ページ遷移
app.py実行後にhttp://localhost:5000/にアクセスするとログイン画面が表示されるので、設定したユーザー名とパスワードを入力してください。
ログインに成功するとwelcomeページが表示されます。
セッションでフラグとユーザー名を変数で保持しており、ログアウトするかブラウザを閉じるまではログイン不要でページを閲覧できます。
おすすめ教材
Udemyのこちら講座がとてもわかりやすかったので、Flaskを基礎から学ぶならおすすめです。
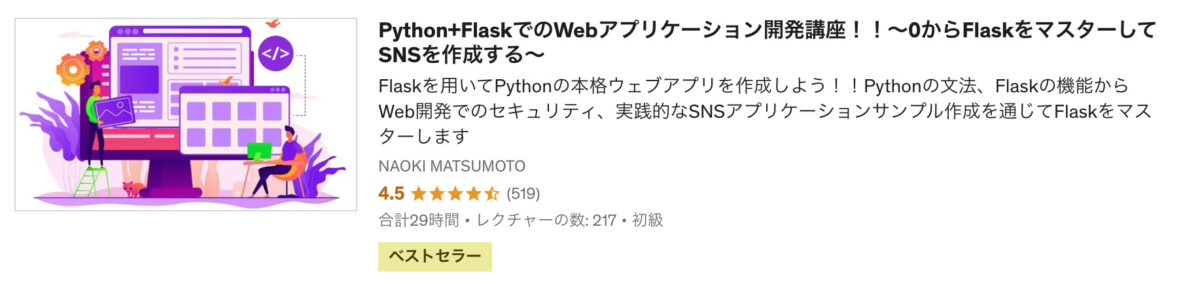